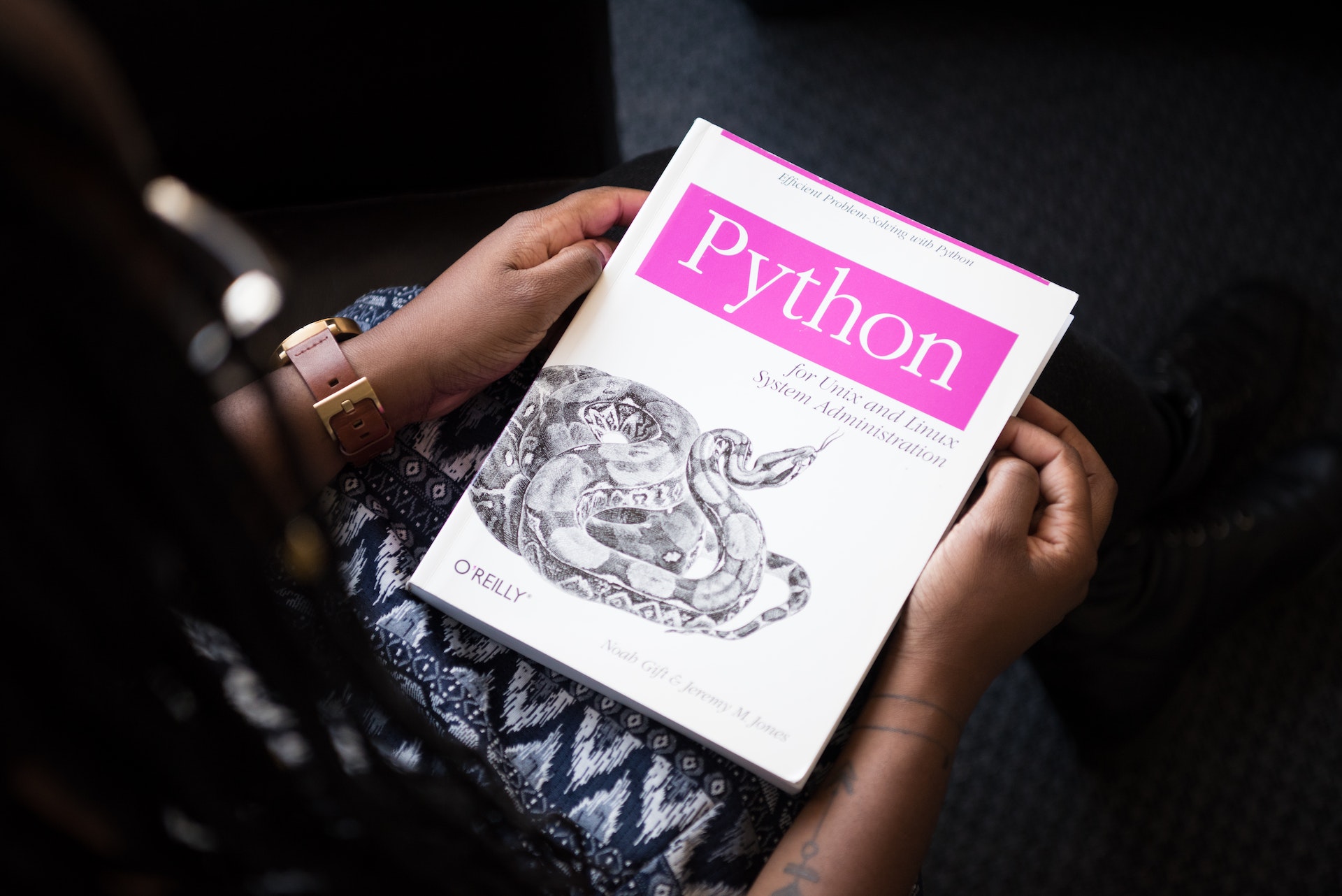
Python, with its versatility, has become a go-to programming language in fields like web development, data analysis, and artificial intelligence. One of its powerful features is the dictionary—a flexible data type that facilitates efficient data management through key-value pairs. Understanding dictionaries is crucial for anyone looking to master Python, as they play a significant role in various real-world applications.
In this blog post, we will provide a comprehensive insight into Python dictionaries, from the basics to advanced techniques, enhancing your programming skill set.
Want to dive deeper into Python? At UpskillsTutor, we connect you with seasoned experts ready to guide your learning journey. Whether you're a beginner or looking to enhance your skills, our tutors are here to help. Elevate your programming journey with personalized guidance—discover what UpskillsTutor has to offer today!
Fundamentals of Python Dictionaries
A Python dictionary is akin to a real-life dictionary, where each word (key) has an associated definition (value). This fundamental characteristic of storing data as key-value pairs makes dictionaries incredibly potent for a multitude of tasks. The keys act as unique identifiers, and the values hold the actual data, enabling a straightforward way to store and access information.
For instance, consider a dictionary to store contact information: contacts = {"John Doe": "[email protected]", "Mary Smith": "[email protected]"}. In this simple example, names serve as keys, and email addresses as values.
It’s this structure that allows for efficient retrieval of data, providing a clear advantage over other data types, such as lists or tuples, especially when dealing with large datasets or when the data structure's key is an intuitive identifier. Dictionaries offer a blend of practicality and efficiency, pivotal for anyone looking to solve real-world problems using Python.
Creating and Modifying Dictionaries
Getting started with Python dictionaries is straightforward. They can be created by enclosing comma-separated key-value pairs within curly braces {}, like so: my_dict = {"key1": "value1", "key2": "value2"}. Alternatively, you can create an empty dictionary using my_dict = dict() and populate it later. Adding new entries is as easy as specifying a new key and assigning a value to it: my_dict["key3"] = "value3".
Modifying a dictionary is equally intuitive; you simply reassign a new value to an existing key. If the key doesn't exist, Python will add it to the dictionary: my_dict["key2"] = "new_value2". Deleting entries is done using the del keyword: del my_dict["key1"].
These basic operations form the cornerstone of interacting with dictionaries, allowing for dynamic data handling. As you become comfortable with them, you'll find that dictionaries are a versatile tool for storing and managing data in your coding projects.
Read more: Learn to Code in Java as a Beginner
Accessing Dictionary Values
Accessing values in a dictionary is a straightforward process, thanks to the key-based indexing it provides. Using the key, you can quickly retrieve the associated value: value = my_dict["key2"].
However, a common hiccup occurs when trying to access a key that doesn’t exist, resulting in a KeyError. To handle such cases gracefully, Python offers the get() method: value = my_dict.get("key2", default_value). This method returns None or a default_value if the key is missing, preventing potential runtime errors.
Moreover, it's good practice to check for key existence before accessing its value, which can be done using the in keyword: if "key2" in my_dict: value = my_dict["key2"]. These safeguards ensure the smooth operation of your code, making dictionary value access reliable and error-free.
Read more: How To Learn HTML
Advanced Dictionary Techniques
As you delve deeper into Python, exploring advanced dictionary techniques can be a game changer in handling complex data scenarios. Dictionary comprehensions, akin to list comprehensions, offer a concise way to create and modify dictionaries: {k: v for k, v in iterable}. Additionally, merging dictionaries has been simplified in Python 3.9 with the advent of the union operators | and |=: merged_dict = dict1 | dict2.
Other powerful methods include items(), keys(), and values() which provide iterable views of a dictionary’s key-value pairs, keys, and values respectively. For instance, for key, value in my_dict.items(): allows you to iterate through all key-value pairs in the dictionary.
Leveraging these advanced techniques can significantly optimize your code, making your data-handling tasks more efficient and readable. Learning these techniques, possibly under the guidance of seasoned tutors, can help you tackle real-world problems with a refined and sophisticated approach, elevating your Python proficiency to a higher echelon.
Python Dictionaries for Real-world Applications
Python dictionaries are not just theoretical constructs; they are powerful tools that are often used in the real world. From managing user data in web applications to configuring settings in software, dictionaries prove indispensable.
Consider data analytics: dictionaries help in aggregating results, mapping unique identifiers to respective data points, or even transforming datasets for further processing.
This means that mastering dictionaries is not an end in itself, but a means to solve practical problems efficiently. Whether you're developing a complex application or simplifying a repetitive task, a deep understanding of dictionaries can be transformative.
Summing Up
Understanding Python dictionaries is an important step in your programming journey. This unique data structure offers a blend of simplicity and efficiency, providing a robust platform for tackling real-world problems.
As you become proficient in creating, modifying, and leveraging dictionaries, you'll discover they are indispensable tools in your coding arsenal. The knowledge you gain will not only enhance your problem-solving capabilities but also provide a solid foundation for exploring more advanced Python concepts.
So, delve into the world of dictionaries, experiment with different techniques, and unlock a new level of programming prowess.